Procedural Terrain Generation
Using Poisson Disc Sampling
Introduction
Creating a terrain manually for a game or just for visualization purposes is a really daunting task. Instead, a better way to do so is to programmatically generate it by passing some parameters to a code. Here, I discuss how to make a procedural terrain generator mostly using processing for python. This can also be done in any other language or game engine or 3D design software. I will be making a simple terrain using height maps for stylized purposes. But, first we need to understand how to generate height maps using perlin noise.
Perlin noise is a procedurally generated noise texture developed by ken perlin. How perlin noise is generated using code is discussed thoroughly here. I’ll be using the built in noise function that generates perlin noise for processing.
Generating sample points
In order to render the terrain, we need some geometry. We can generate tile the plane with cubes, but that would look more like minecraft. We could choose randomly generated points. But purely random or even pseudo-random choices have a tendency of clustering together. This phenomenon is known as Poisson Clumping
. Pure or pseudo-random points are not good choices because of this phenomenon, as shown below.
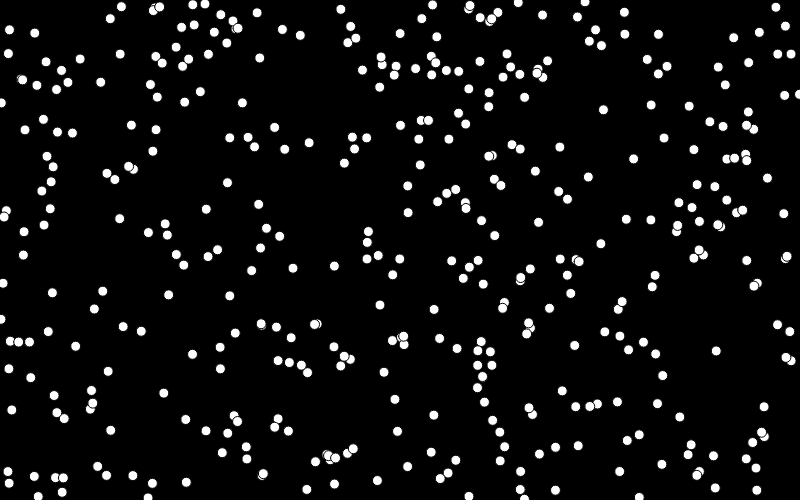
So, instead we use a different sort of random point generation called Poisson Disc Sampling
. This generates random points at a given interval and thus making them more uniform. This is a much better choice for generating terrains.
Now if we triangulate the points,We should get a plane with enough geometry to offset using the generated height map. I go into some details on triangulation here
. The final result should look something like this (without the animation of course):
Creating a rough 3D terrain
Now we rotate the canvas on the y-axis so it looks more like a plane.
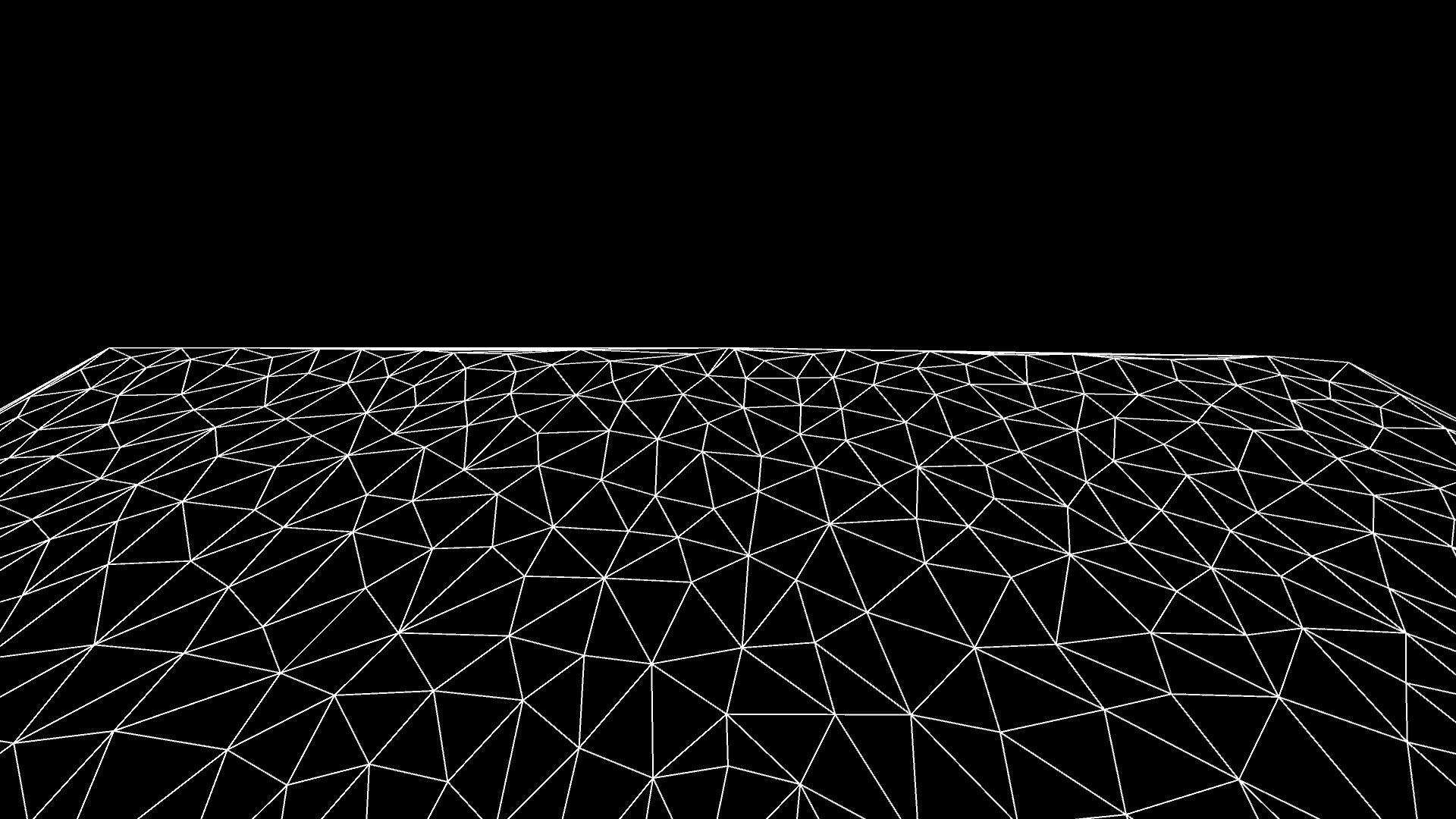
Now, using the generated height map, we offset the z-position of the points by the value corresponding to that x and y position in the height map. This gives us a stylized terrain.
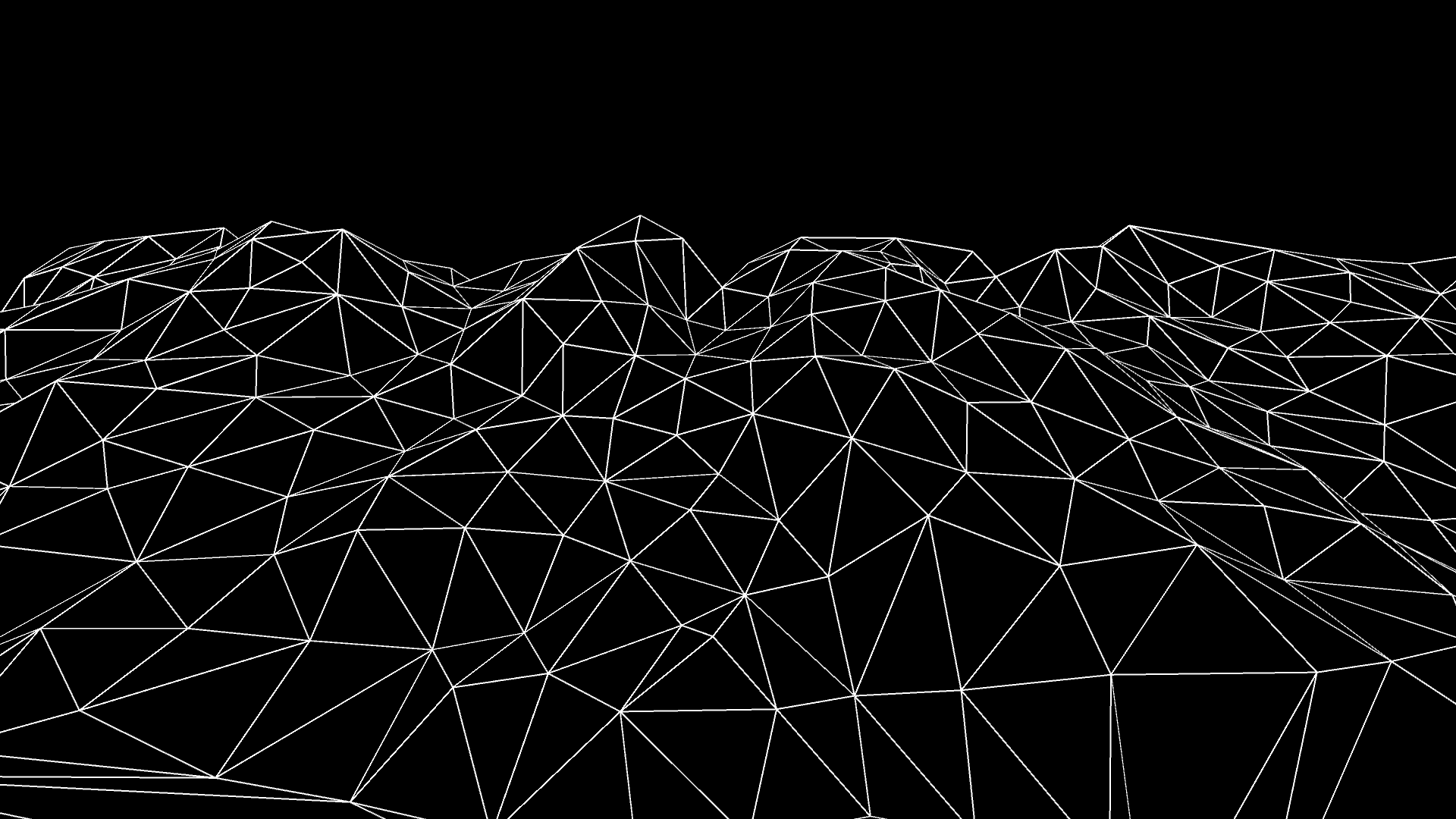
We can change the shape of this terrain by changing the noise using offsets. This can result in traversing through the terrain as if we were flying on top of it, or changing the entire topology of the terrain. It will depend on how the noise is being offset.
I’ve put the processing.py codes for this project here
.
Smoother surfaces
The generated surface is still pretty low resolution and looks pointy. This can be solved in two ways. First, we can either increase the number of points from the poisson disk sampling process. The other method is using the Catmull-Clark algorithm
. This is a technique used in 3D computer graphics to represent curved surfaces by the specification of a coarser polygonal mesh and produced by a recursive algorithmic method.
Furthermore, the same process can be used to generate terrain in 3D modelling software. The Catmull-Clark algorithm can be used easily here as most software have built in support. The following demonstrates the improvements made while using the algorithm.
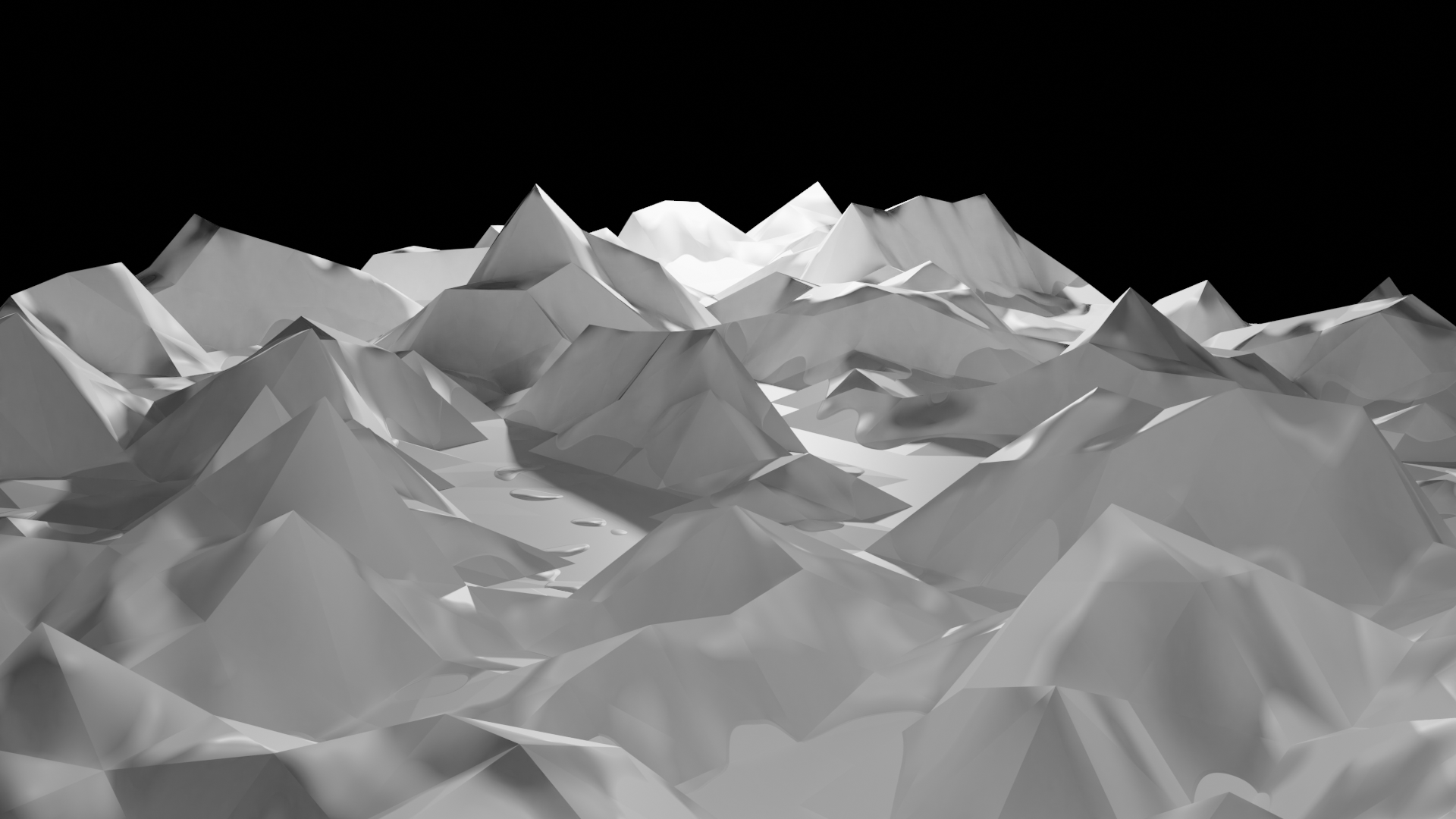
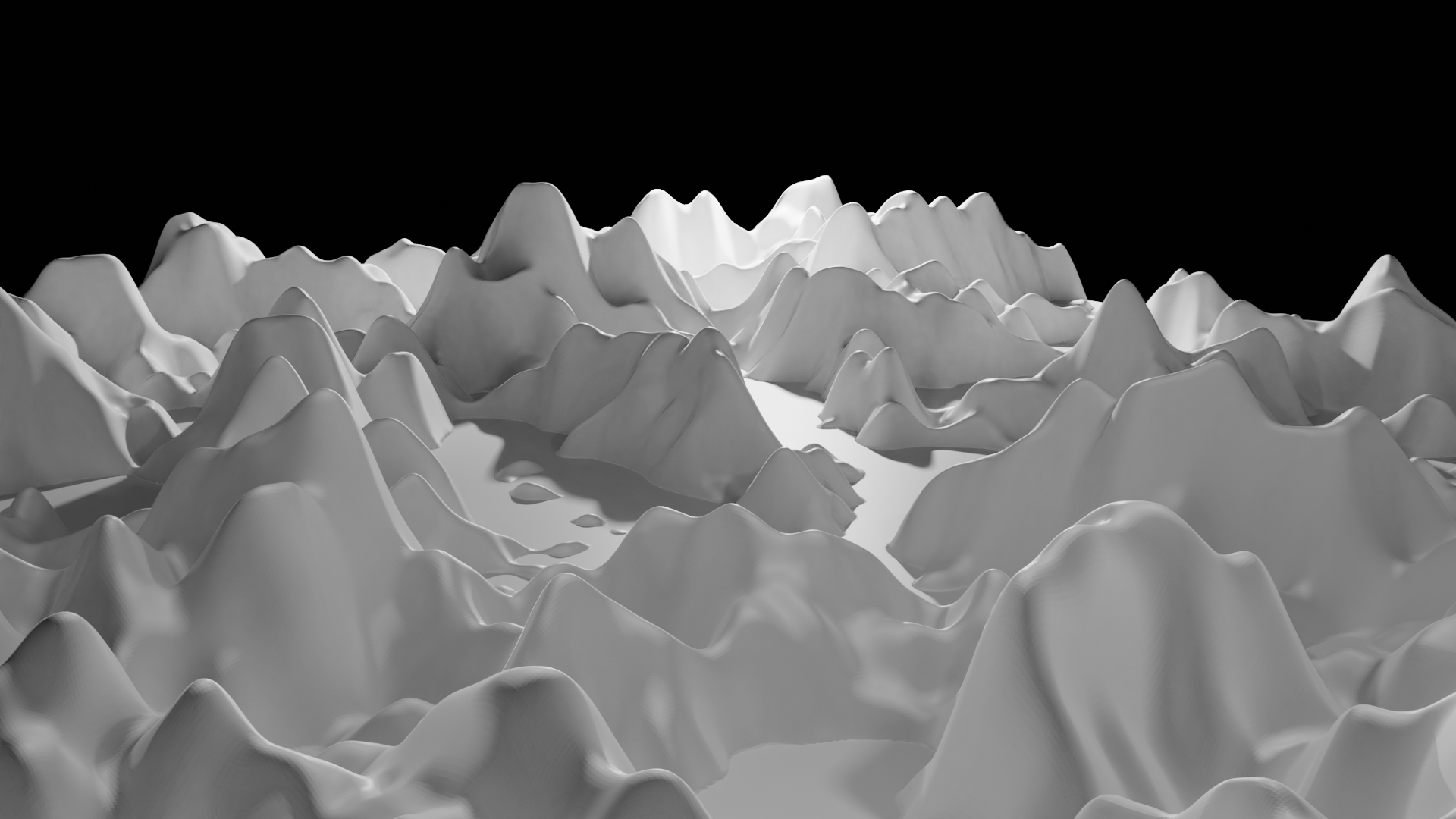
Example videos
Below are two different renders I’ve made using the processing.py. One with a half inverted render and the other colored according to the height of the points i.e. a topological height map.